Introducing Restless
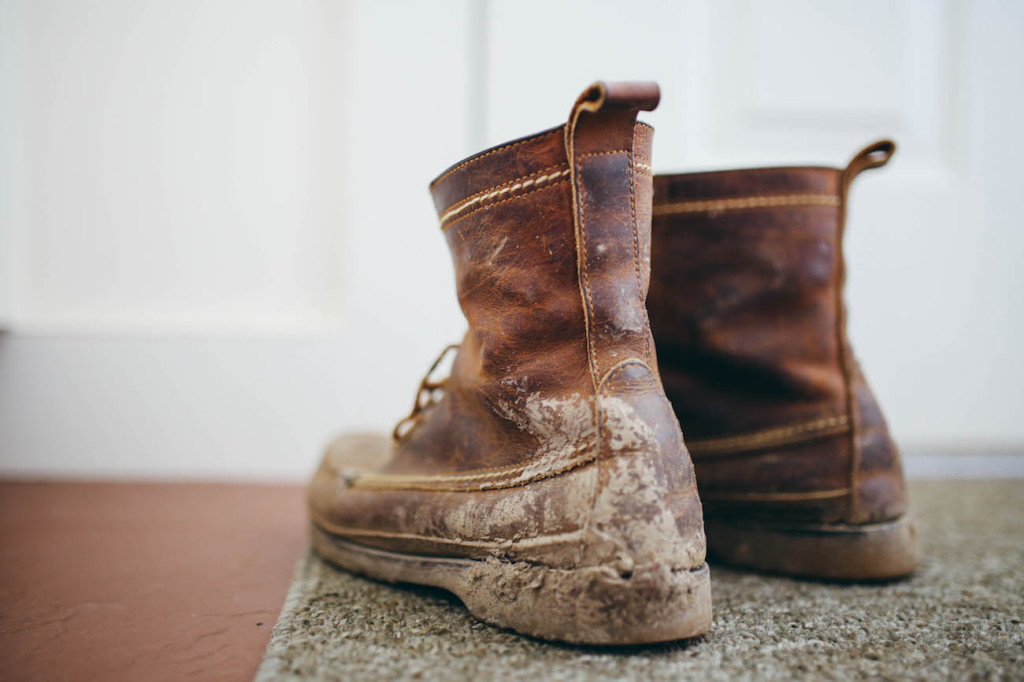
For a while now, in my “spare time”, I’ve been working on a new library for iOS. And I’m pleased to announce that it is now ready for public consumption.
Introducing Restless, “a type-safe HTTP client for iOS and OS X”. If you’re familiar with the excellent Retrofit library for Android, that tagline may sound familiar. In fact Restless is inspired by, and heavily modeled, on Retrofit.
If you don’t already know Retrofit, here’s the basic idea: instead of writing all the network calls (and their associated boilerplate code) manually, you just define a protocol that describes the functions of the web service. And you use annotations to describe how each method should work. Something like this:
@protocol GitHubService <DRWebService>
@GET("/users/{user}/repos")
- (NSURLSessionDataTask*)listRepos:(NSString*)user
callback:DR_CALLBACK(NSArray<GitHubRepo*>*)callback;
@POST("/users/new")
@Body("user")
- (NSURLSessionUploadTask*)createUser:(User*)user
callback:DR_CALLBACK(User*)callback;
@end
Then at runtime, you ask Restless to build you an implementation of the protocol:
DRRestAdapter* ra = [DRRestAdapter restAdapterWithBlock:^(DRRestAdapterBuilder *builder) {
builder.endPoint = [NSURL URLWithString:@"https://api.github.com"];
}];
NSObject* service = [ra create:@protocol(GitHubService)];
Now it’s easy to call those methods:
NSURLSessionDataTask* task = [service listRepos:@"natep"
callback:^(NSArray<GitHubRepo*>* result,
NSURLResponse *response,
NSError *error)
{
// response handling ...
}];
[task resume];
And Restless will automatically convert the results into the type you asked for in the callback parameter. So you’ve got a readable, centralized definition of how your web service works, and all the ugly bits are encapsulated in the dynamic implementation that Restless creates.
This is just the basic overview. If you want to know more, please check out the Github repo.